Day 2: Understanding Containers
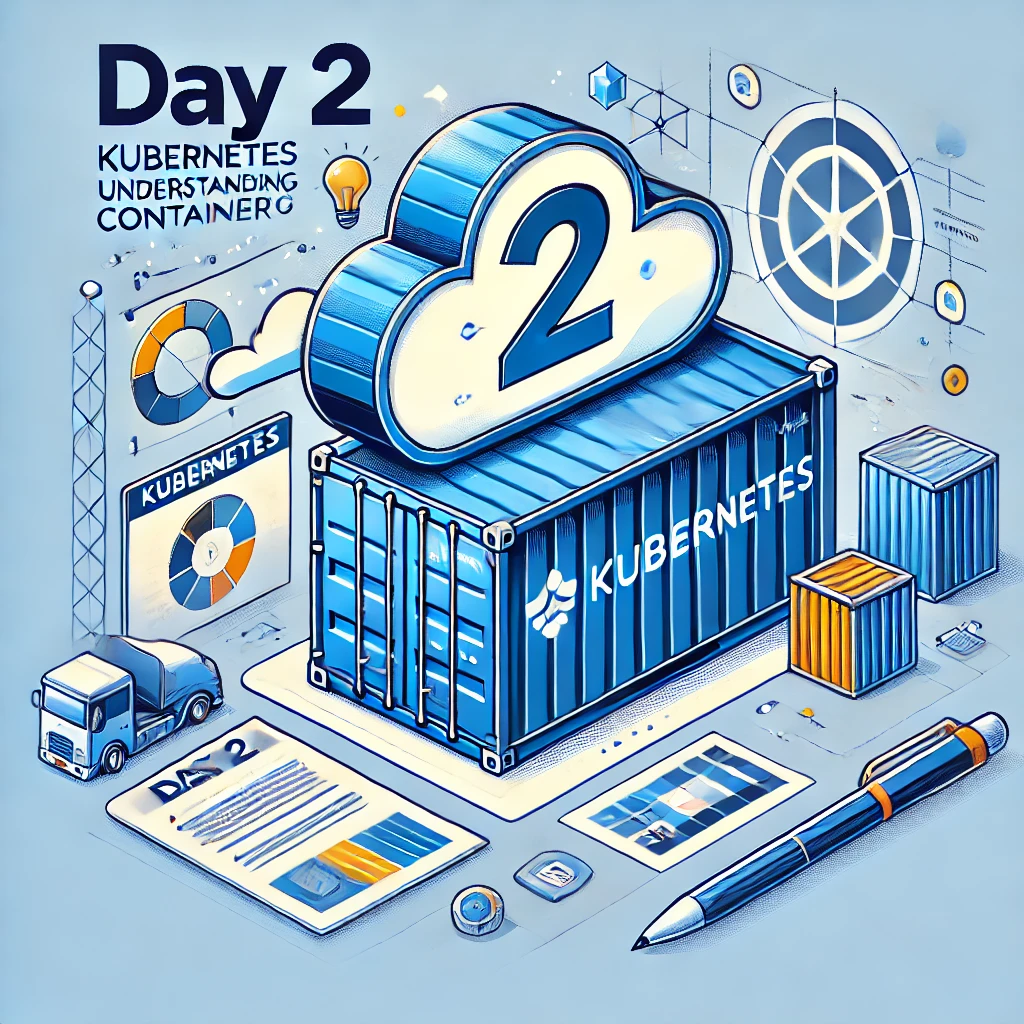
What Are Containers?
Containers are lightweight, portable, and self-sufficient environments that encapsulate an application and all its dependencies. Unlike virtual machines (VMs), containers share the host operating system kernel, making them more efficient and faster to start. Containers ensure consistency across different environments—from development to testing to production.
Key Characteristics of Containers:
- Lightweight: Containers are smaller in size compared to VMs as they share the host OS kernel.
- Portable: Run anywhere—on-premise, cloud, or hybrid environments.
- Isolated: Each container operates independently, ensuring no interference with other containers.
- Efficient: Optimized resource utilization due to shared OS kernel.
Basics of Docker
Docker is the most popular platform for creating, deploying, and managing containers. It simplifies containerization by providing tools to build and run container images. Docker’s widespread adoption has made it the standard for containerized application development.
Key Docker Components:
- Docker Images: Read-only templates to create containers.
- Docker Containers: Running instances of Docker images.
- Docker Engine: The runtime responsible for building and running containers.
- Docker Hub: A public repository for sharing and storing images.
Installing Docker
Before building your first container, you need to have Docker installed. Follow these steps:
1. Install Docker on Linux:
# Update the package database
sudo apt-get update
# Install required packages
sudo apt-get install apt-transport-https ca-certificates curl software-properties-common
# Add Docker’s GPG key
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo apt-key add -
# Add Docker repository
sudo add-apt-repository \
"deb [arch=amd64] https://download.docker.com/linux/ubuntu \
$(lsb_release -cs) \
stable"
# Install Docker
sudo apt-get update
sudo apt-get install docker-ce docker-ce-cli containerd.io
# Verify Docker installation
sudo docker --version
2. Install Docker on macOS or Windows:
Download Docker Desktop from Docker’s Official Website. Follow the installation instructions specific to your operating system.
Building and Running Your First Container
Now that Docker is installed, let’s build and run a simple container.
Step 1: Create a Dockerfile
A Dockerfile is a script with instructions to build a Docker image.
# Use an official Node.js runtime as the base image
FROM node:16
# Set the working directory in the container
WORKDIR /usr/src/app
# Copy package.json and install dependencies
COPY package.json .
RUN npm install
# Copy application files
COPY . .
# Expose port 3000
EXPOSE 3000
# Start the application
CMD [ "node", "app.js" ]
Step 2: Build the Docker Image
Run the following command to create a Docker image:
# Build the image and tag it as "my-node-app"
docker build -t my-node-app .
Step 3: Run the Docker Container
Once the image is built, run it as a container:
# Run the container on port 3000
docker run -p 3000:3000 my-node-app
You should now be able to access your application on http://localhost:3000
.
Benefits of Using Containers
- Consistency Across Environments: Containers package applications and their dependencies together, eliminating the “it works on my machine” problem.
- Faster Development Cycles: Containers can be started and stopped quickly, enabling rapid iteration.
- Improved Scalability: Containers can be replicated easily to handle increased load.
- Simplified Deployment: With containers, deploying to production becomes as simple as running a command.
Common Docker Commands
Here are some essential Docker commands to get you started:
# List running containers
docker ps
# List all containers (including stopped ones)
docker ps -a
# Stop a running container
docker stop <container_id>
# Remove a container
docker rm <container_id>
# Remove an image
docker rmi <image_id>
Conclusion
Understanding containers and Docker is fundamental to working with Kubernetes. Containers form the backbone of Kubernetes’ functionality. By mastering Docker basics, you’re building a solid foundation for the rest of this 30-day learning journey.
References
*** Your support will help me continue to bring new Content. Love Coding ***❤️
Feedback and Discussion
Have questions or feedback? Comment below! Let’s build a collaborative learning environment. Check out more articles on Node.js, Express.js, and System Design.