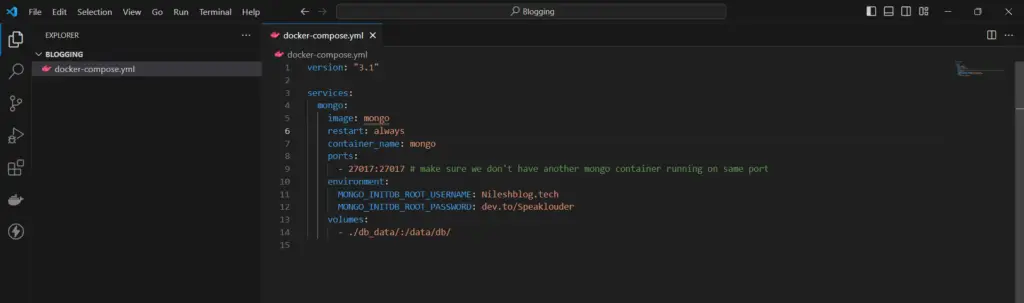
How to Use Large Language Models (LLMs) to Build Projects
Large Language Models (LLMs) have emerged as powerful tools for various applications, from chatbots to content generation. Whether you’re interested in developing AI-powered applications, automating workflows, or generating revenue through innovative solutions, LLMs provide incredible opportunities. This guide will help students with limited technical knowledge understand LLMs, how they work, and how to use them in real-world projects.
1. What Are Large Language Models (LLMs)?
LLMs are a type of artificial intelligence (AI) designed to understand and generate human language. They are trained on vast amounts of text data from the internet, books, and other sources, enabling them to predict words and generate meaningful responses. Popular LLMs like OpenAI’s GPT (Generative Pretrained Transformer) and Google’s Gemini model are capable of tasks like answering questions, writing essays, and even coding.
Think of an LLM as a highly advanced version of predictive text on your phone — it doesn’t just guess the next word, but can create entire paragraphs, respond to questions, and solve problems.
2. How Do LLMs Work?
LLMs use a technology called deep learning, specifically a type of neural network architecture known as the Transformer. They process large amounts of text to learn grammar, facts, and the relationships between words, phrases, and concepts.
Here’s a simplified explanation of how LLMs generate language:
- Training: LLMs are trained by feeding them vast datasets of text (e.g., books, websites). They learn patterns, relationships, and facts from this data.
- Tokenization: When you input a sentence, the model breaks it into smaller pieces called “tokens” (words or parts of words).
- Prediction: The model predicts the next token based on the previous ones. By repeating this process, it generates a coherent response.
3. Why Should You Learn LLMs?
LLMs can revolutionize various fields such as education, healthcare, entertainment, and business. By learning how to use LLMs, you can:
- Build real-world applications: Create chatbots, language translators, or automated writing tools.
- Improve your learning experience: Use LLMs to generate study materials, get quick explanations, and improve understanding.
- Unlock business potential: You can monetize your LLM-based applications by offering services or tools that solve real-world problems.
4. Getting Started with LLMs: Tools and Platforms
Even with limited technical knowledge, you can start using LLMs thanks to beginner-friendly platforms. Here are some popular tools:
- OpenAI (GPT Models): OpenAI provides API access to their GPT models. You can easily integrate these into your projects by making API calls. You can start by signing up at OpenAI.
- Google’s Gemini: Another powerful model that provides text-generation capabilities. You can explore Google’s APIs through the Google Cloud platform.
- Hugging Face: Hugging Face hosts a wide range of LLMs and provides an easy-to-use interface for beginners. Check it out at Hugging Face.
- LangChain: A framework that helps developers create LLM-powered applications using Python.
Most platforms provide free access for small-scale projects, so you won’t need a budget to start learning.
5. Building Simple Projects with LLMs
Let’s explore how to create three basic projects using LLMs. You’ll need an API key from a platform like OpenAI or Hugging Face. You can use JavaScript or Python to make API calls, but even if you don’t know how to code, you can still explore some low-code options.
Project 1: Building a Chatbot
A chatbot is a simple, interactive program that can have conversations with users. Here’s how you can build one:
- Sign up for OpenAI API: Get an API key from OpenAI.
- Write a simple script: Using Python or JavaScript, write a script to send user input to the GPT model and return a response.
Here’s an example in Python:
import openai
openai.api_key = "your-api-key"
def chatbot():
while True:
user_input = input("You: ")
if user_input.lower() == 'quit':
break
response = openai.Completion.create(
engine="text-davinci-003",
prompt=user_input,
max_tokens=150
)
print("Bot: " + response.choices[0].text.strip())
chatbot()
This code sends your message to the GPT model and returns a chatbot response. You can improve it by creating a user-friendly interface.
Project 2: Content Generation Tool
LLMs can generate creative content such as blog posts, social media captions, or even poems. Here’s a simple way to build a content generation tool:
- Get an API key from OpenAI or Hugging Face.
- Build a web form: Create an input field where users can enter a topic or keywords.
- Generate content: Send the input to the API and return a piece of content.
import openai
openai.api_key = "your-api-key"
def generate_content(topic):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=f"Write a blog post about {topic}",
max_tokens=300
)
return response.choices[0].text.strip()
topic = input("Enter a topic: ")
print(generate_content(topic))
You can build this into a web app or mobile app, and users can generate content based on any topic.
Project 3: AI Tutor for Students
You can build an AI tutor that helps students with their homework by providing explanations and solutions to problems.
- Create a subject list: Allow users to select subjects like math, science, etc.
- Ask for specific questions: The user enters a question.
- Generate the answer: The LLM will provide a detailed explanation.
Example in Python:
import openai
openai.api_key = "your-api-key"
def ai_tutor(question):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=f"Explain the answer to the following question: {question}",
max_tokens=200
)
return response.choices[0].text.strip()
question = input("Enter your question: ")
print(ai_tutor(question))
6. Advanced Projects for Students with Some Coding Knowledge
Once you’re comfortable with the basics, you can move on to more complex projects.
Project 4: Automating Tasks with LLMs
You can automate repetitive tasks, such as drafting emails or generating reports. For example, you could write a script that automatically drafts a professional email based on a few keywords.
import openai
def draft_email(subject, recipient, body_keywords):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=f"Draft a formal email to {recipient} about {subject} using the following keywords: {body_keywords}",
max_tokens=150
)
return response.choices[0].text.strip()
subject = "Meeting Follow-up"
recipient = "John Doe"
body_keywords = "next steps, project update"
print(draft_email(subject, recipient, body_keywords))
Project 5: Summarizing Text and Documents
LLMs can summarize long documents, making them valuable tools for research and study. You can create a tool that summarizes articles, PDFs, or essays.
- Input a URL or document text.
- Send the text to the LLM for summarization.
- Display the summary.
def summarize_text(text):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=f"Summarize the following text: {text}",
max_tokens=100
)
return response.choices[0].text.strip()
text = "Your document or article text here..."
print(summarize_text(text))
7. Potential Revenue Streams from LLM Projects
After building some LLM-powered projects, you can monetize your skills and solutions:
- Freelancing: Offer AI services like content generation, chatbot development, or automation tools to businesses through platforms like Upwork or Fiverr.
- Subscription-based Tools: Create a web app (e.g., a content generator or AI tutor) and charge users a subscription fee.
- AI-Powered Products: Develop apps or tools that help businesses or individuals save time,
and offer them as products.
4. Consulting: Provide LLM integration services to companies looking to adopt AI in their workflows.
8. Final Tips for Learning LLMs
- Practice: Experiment with different APIs and create small projects.
- Join communities: Participate in online forums, GitHub, or Discord channels to learn from others.
- Follow tutorials: Explore free resources and courses from platforms like Coursera, Udemy, or YouTube to deepen your knowledge.
9. Resources for Further Learning
- OpenAI Documentation: OpenAI API Documentation
- Hugging Face Tutorials: Hugging Face Tutorials
- YouTube Channels: Check out AI-focused YouTube channels like Two Minute Papers or Sentdex.
Conclusion
Large Language Models (LLMs) are transforming the way we interact with technology. With just a basic understanding of LLMs, you can create powerful applications like chatbots, content generators, and AI tutors. Whether you’re a student looking to boost your learning or an aspiring entrepreneur, LLMs offer endless possibilities for innovation and income. The key is to start small, practice consistently, and expand your knowledge step by step. Happy learning!
This article should be helpful for beginners who are excited to dive into the world of LLMs and build meaningful projects.