LeetCode 132. Pattern: Hey there, coding enthusiasts! Welcome back to another exciting coding session. Today’s problem is a treat—literally! We’re going to solve the “pattern” or “LeetCode .132”
Stack Approach: Leet Code 132. Pattern (Medium)
- Define a Stack: Create a stack to keep track of potential “132 pattern” candidates.
- Initialize Variables: Initialize a variable “third” to store the third element in the pattern with the minimum possible value.
- Iterate in Reverse: Start iterating through the given array in reverse order.
- Check for Pattern: If the current number is less than “third,” it means we found a “132 pattern,” and we return true.
- Update Third Element: While the stack is not empty and the top element of the stack is less than the current number, update “third” with the top element and pop it from the stack.
- Push to Stack: Push the current number onto the stack for further evaluation.
- Return False: If no “132 pattern” is found, return false.
C++: Stack Leet Code 132
class Solution {
public:
bool find132pattern(vector<int>& nums) {
int length = nums.size();
if (length < 3)
return false;
stack<int> stack;
int third = INT_MIN;
for (int i = length - 1; i >= 0; i--) {
int ele=nums[i];
if (ele < third)
return true;
while (!stack.empty() && stack.top() <ele ) {
third = stack.top();
stack.pop();
}
stack.push(ele);
}
return false;
}
};
Java: Stack Leet Code 132
public class Solution {
public boolean find132pattern(int[] nums) {
int length= nums.length;
if (length < 3)
return false;
Deque<Integer> stack = new ArrayDeque<>(length);
int third = Integer.MIN_VALUE;
for (int i = length - 1; i >= 0; i--) {
if (nums[i] < third) return true;
while (!stack.isEmpty() && stack.peek() < nums[i]) {
third = stack.pop();
}
stack.push(nums[i]);
}
return false;
}
}
Python: Stack LeetCode 132
class Solution:
def find132pattern(self, nums: List[int]) -> bool:
length = len(nums)
if length < 3:
return False
stack = deque()
third = float('-inf')
for i in range(length - 1, -1, -1):
num=nums[i]
if num < third:
return True
while stack and stack[0] < num:
third = stack.popleft()
stack.appendleft(num)
return False
JavaScript: Stack Leet Code 132
/**
* @param {number[]} nums
* @return {boolean}
*/
var find132pattern = function(nums) {
const stack = [];
let third = Number.NEGATIVE_INFINITY;
for (let i = nums.length - 1; i >= 0; i--) {
if (nums[i] < third) {
return true;
}
while (stack.length > 0 && stack[stack.length - 1] < nums[i]) {
third = stack.pop();
}
stack.push(nums[i]);
}
return false;
};
Result Analysis
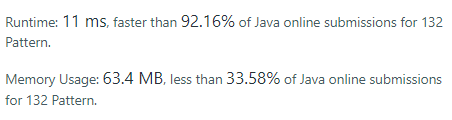
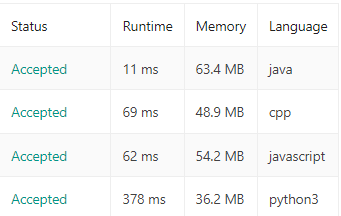
List of Some important Leet code Questions:
- Leet Code 835. Image Overlap
- Leet Code 662. maximum width of binary tree
- Leet Code 287 Find the Duplicate Number
- Leetcode 135 Candy (Hard) Solution
- Leet Code 2612 Minimum Reverse Operations
- Leet code 206 Reverse Linked List
- Leet Code 420 Strong Password Checker
- Leetcode 1359 Count All Valid Pickup and Delivery
- Leet code 799. Champagne Tower
- LeetCode 389. Find The Difference
- Leetcode 775. Find The Global and Local Inversions