Leetcode 389. Find The Difference :Hey there, coding enthusiasts! Welcome back to another exciting coding session. Today’s problem is a treat—literally! We’re going to solve the “Find The Difference ” problem.
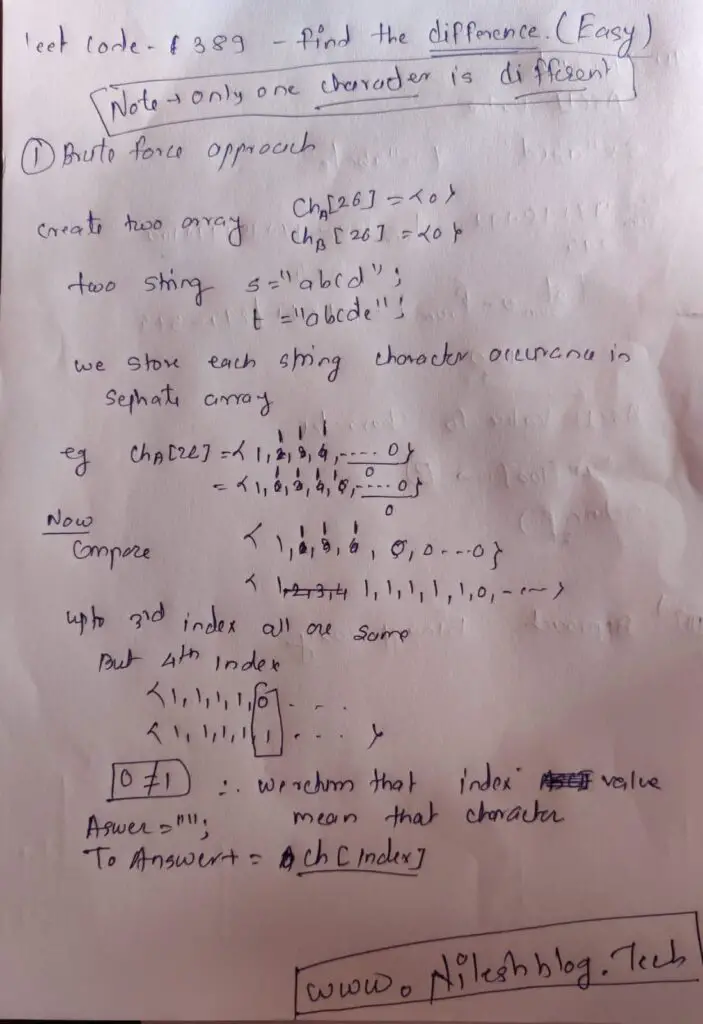
Bruteforce Approach
1: Understand the Problem
- In this problem, we’re given two strings, one of which is formed by adding a single character to the other string. Our task is to find that additional character.
- Think of it as trying to spot a new letter that was added to one of the strings.
2: Read the Input
- We have two strings,
s
andt
, wheret
is the string with an extra character. - For example, if
s = "abcd"
andt = "abcde"
, then “e” is the extra character.
3: Count Character Occurrences
- Create two arrays or dictionaries to count the occurrences of each character in both strings.
- Loop through
s
and count how many times each character appears, and do the same fort
.
4: Compare the Counts
- Compare the character counts between the two strings.
- Look for a character that has a different count in
t
compared tos
. This character will be the additional one.
5: Return the Result
- Once you find the differing character, return it as the result.
Codes: Leetcode 389 find the difference bruteforce
C++ : bruteforce Approach
#include <iostream>
#include <string>
class Solution {
public:
char findTheDifference(std::string s, std::string t) {
int count1[26] = {0};
int count2[26] = {0};
for (char c : s) {
count1[c - 'a']++;
}
for (char c : t) {
count2[c - 'a']++;
}
for (int i = 0; i < 26; i++) {
if (count1[i] != count2[i]) {
return 'a' + i;
}
}
return '\0'; // If no difference is found.
}
};
Leet Code : Longest Palindromic Substring Java | CPP | Java Script | Python Solution
Java : bruteforce Approach
public class Solution {
public char findTheDifference(String s, String t) {
int[] count1 = new int[26];
int[] count2 = new int[26];
for (char c : s.toCharArray()) {
count1[c - 'a']++;
}
for (char c : t.toCharArray()) {
count2[c - 'a']++;
}
for (int i = 0; i < 26; i++) {
if (count1[i] != count2[i]) {
return (char)('a' + i);
}
}
return '\0'; // If no difference is found.
}
}
Leet Code: Longest Substring Without Repeating Characters Java | JavaScript | CPP | Python Solution
Python : bruteforce Approach
class Solution:
def findTheDifference(self, s, t):
count1 = [0] * 26
count2 = [0] * 26
for c in s:
count1[ord(c) - ord('a')] += 1
for c in t:
count2[ord(c) - ord('a')] += 1
for i in range(26):
if count1[i] != count2[i]:
return chr(ord('a') + i)
return None # If no difference is found.
Find the Longest Substring Without Repeating Characters: Solution and Algorithm
JavaScript : bruteforce Approach
class Solution {
findTheDifference(s, t) {
const count1 = new Array(26).fill(0);
const count2 = new Array(26).fill(0);
for (const c of s) {
count1[c.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
for (const c of t) {
count2[c.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
for (let i = 0; i < 26; i++) {
if (count1[i] !== count2[i]) {
return String.fromCharCode('a'.charCodeAt(0) + i);
}
}
return null; // If no difference is found.
}
}
Leet code :Zigzag Conversion solution c++ , Java , Python , JavaScript , Swift ,TypeScript
Ascii Value Approach: Leetcode 389 find the
- It is the simplest approach, we take individual string, find the Ascii Value of each character, and then sum up all values.
- same for Anthor String.
- Take difference between the ,value we find (|Ascii sum of t – Ascii sum of s|)
- return the character contain that Remaining Ascii value ((|Ascii sum of t – Ascii sum of s|)).
- return chacter[Ascii sum of t – Ascii sum of s];
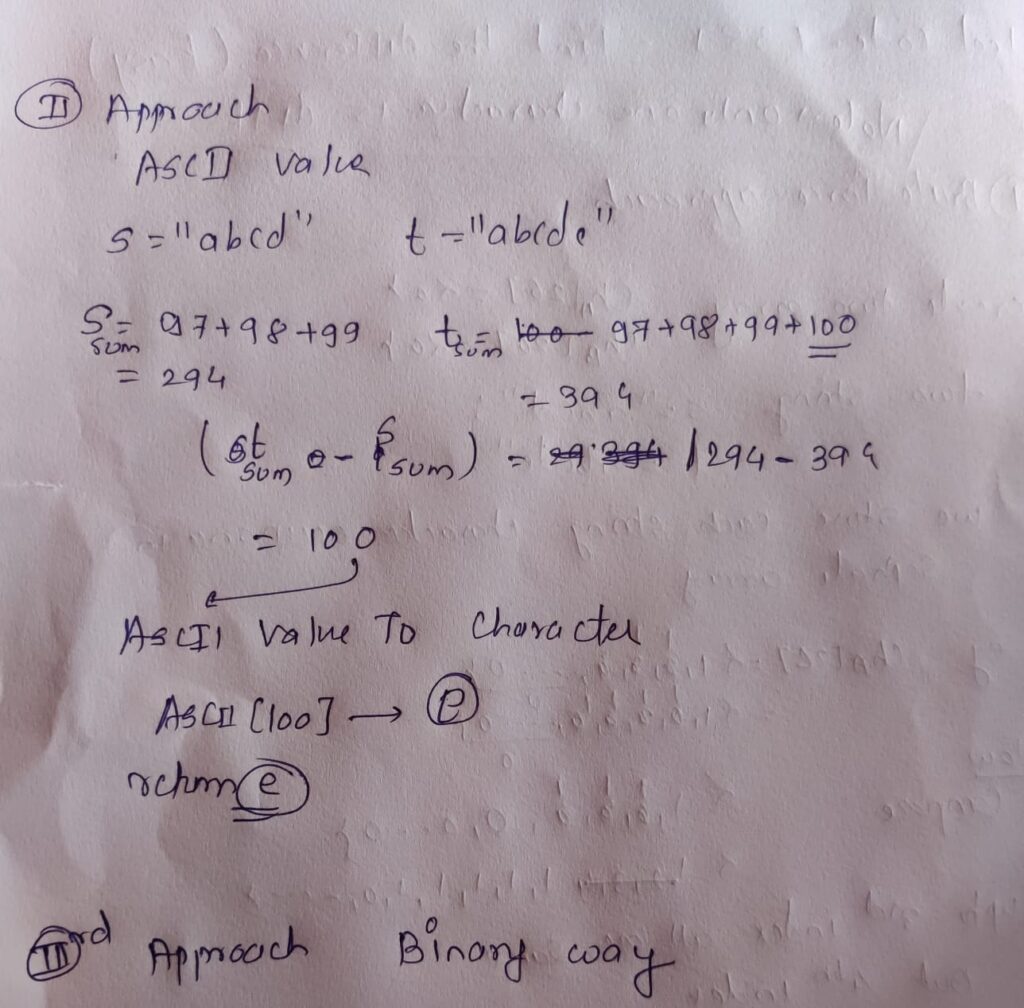
Java: Ascii Value Approach
public class Solution {
public char findTheDifference(String s, String t) {
for (int i = 0; i < s.length(); i++) {
t = t.substring(0, i + 1) + (char) (t.charAt(i) - s.charAt(i)) + t.substring(i + 1);
}
return t.charAt(t.length() - 1);
}
}
LeetCode: A Quick and Simple Solution to Find the Town Judge
Python: Ascii Value Approach
class Solution:
def findTheDifference(self, s: str, t: str) -> str:
for i in range(len(s)):
t = t[:i+1] + chr(ord(t[i]) - ord(s[i)) + t[i+1:]
return t[-1]
JavaScript: Ascii Value Approach
class Solution {
findTheDifference(s, t) {
for (let i = 0; i < s.length; i++) {
t = t.substring(0, i + 1) + String.fromCharCode(t.charCodeAt(i) - s.charCodeAt(i)) + t.substring(i + 1);
}
return t.charAt(t.length - 1);
}
}
List of Some important Leet code Question :
- Leet Code 835. Image Overlap
- Leet Code 662. maximum width of binary tree
- Leet Code 287 Find the Duplicate Number
- Leetcode 135 Candy (Hard) Solution
- Leet Code 2612 Minimum Reverse Operations
- Leet code 206 Reverse Linked List
- Leet Code 420 Strong Password Checker
- Leetcode 1359 Count All Valid Pickup and Delivery
- Leet code 799. Champagne Tower