LeetCode 557. Reverse Words in a String III Hey there, coding enthusiasts! Welcome back to another exciting coding session. Today’s problem is a treat—literally! We’re going to solve the “Reverse Words in a String III ” or “LeetCode .557‘
1 Appraoch : Simple (Brute Force) :Reverse Words in a String
- Initialize variables and iterate through the input string.
- When a word is found (either space or end of string), reverse it in-place.
- Update the ‘start’ pointer for the next word.
- Return the modified string.
Codes: Bruteforce Reverse Words in a String
Certainly! Here’s the code to reverse words in a string using a two-pointer approach in C++, Java, Python, and JavaScript:
C++:Bruteforce
#include <algorithm>
class Solution {
public:
string reverseWords(string s) {
int n = s.length();
int start = 0;
for (int i = 0; i <= n; ++i) {
if (i == n || s[i] == ' ') {
reverse(s.begin() + start, s.begin() + i);
start = i + 1;
}
}
return s;
}
};
Java:Bruteforce
public class Solution {
public String reverseWords(String s) {
char[] chars = s.toCharArray();
int n = chars.length;
int start = 0;
for (int i = 0; i <= n; ++i) {
if (i == n || chars[i] == ' ') {
reverseWord(chars, start, i - 1);
start = i + 1;
}
}
return new String(chars);
}
private void reverseWord(char[] chars, int left, int right) {
while (left < right) {
char temp = chars[left];
chars[left] = chars[right];
chars[right] = temp;
left++;
right--;
}
}
}
Python: Bruteforce
class Solution:
def reverseWords(self, s: str) -> str:
s = list(s)
n = len(s)
start = 0
for i in range(n + 1):
if i == n or s[i] == ' ':
self.reverseWord(s, start, i - 1)
start = i + 1
return ''.join(s)
def reverseWord(self, s, left, right):
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
JavaScript: Bruteforce
var reverseWords = function(s) {
const chars = s.split('');
const n = chars.length;
let start = 0;
for (let i = 0; i <= n; i++) {
if (i === n || chars[i] === ' ') {
reverseWord(chars, start, i - 1);
start = i + 1;
}
}
return chars.join('');
};
function reverseWord(chars, left, right) {
while (left < right) {
const temp = chars[left];
chars[left] = chars[right];
chars[right] = temp;
left++;
right--;
}
}
2 Approach : Two Pointer : LeetCode 557
1: Initialize Variables
- We begin by initializing some variables. The ‘n’ variable represents the length of the input string ‘s,’ while ‘start’ is a pointer to keep track of the start of each word.
2: Iterate Through the String
- We loop through the characters in the string ‘s,’ using ‘i’ as our iterator.
- We check if we have reached the end of the string or if we’ve encountered a space character (‘ ‘).
- If we do, it means we’ve found a complete word or reached the end of the input.
3: Reverse the Word Using Two Pointers
- When we find a word (either by encountering a space or reaching the end of the string), we use a two-pointer approach to reverse it in-place.
- We have ‘left’ and ‘right’ pointers that initially point to the start and end of the word, respectively.
- While ‘left’ is less than ‘right,’ we swap the characters at these positions using the ‘swap’ function. This efficiently reverses the word in the string.
4: Update the Start Pointer
- After reversing the word, we update the ‘start’ pointer to the position after the space. This prepares us for the next word or the end of the input.
5: Return the Modified String
- Once we’ve processed the entire input string, we return the modified ‘s,’ which now contains the words reversed while preserving whitespace and the original word order.
Codes: Two pointer Reverse Words in a String
C++: Two pointer Reverse Words in a String
class Solution {
public:
string reverseWords(string s) {
int n = s.length();
int start = 0;
for (int i = 0; i <= n; ++i) {
if (i == n || s[i] == ' ') {
int left = start;
int right = i - 1;
while (left < right) {
swap(s[left], s[right]);
left++;
right--;
}
start = i + 1;
}
}
return s;
}
};
Java: Two pointer Reverse Words in a String
public class Solution {
public String reverseWords(String s) {
String[] words = s.split(" ");
StringBuilder result = new StringBuilder();
for (String word : words) {
StringBuilder reversedWord = new StringBuilder(word).reverse();
if (result.length() > 0) {
result.append(" ");
}
result.append(reversedWord);
}
return result.toString();
}
}
Python: Two pointer Reverse Words in a String
class Solution:
def reverseWords(self, s: str) -> str:
words = s.split()
reversed_words = [word[::-1] for word in words]
return ' '.join(reversed_words)
Result Analysis
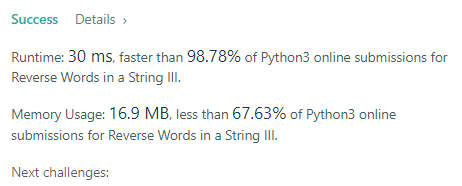
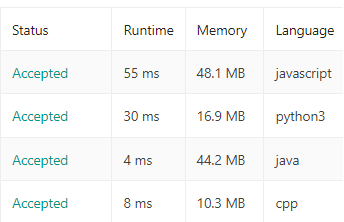
List of Some important Leet code Questions:
- Leet Code 2612 Minimum Reverse Operations
- Leet code 206 Reverse Linked List
- Leet Code 420 Strong Password Checker
- Leetcode 1359 Count All Valid Pickup and Delivery
- Leet code 799. Champagne Tower
- LeetCode 389. Find The Difference
- Leetcode 775. Find The Global and Local Inversions
- Leetcode 316. Remove Duplicate Letters
- LeetCode 2233 Maximum Product After K Increments
- LeetCode 880. Decoded String at Index
- LeetCode 905. Sort Array By Parity
- LeetCode 896. Monotonic Array
- LeetCode 132. Pattern