Leetcode 706. Design HashMap:Hey there, coding enthusiasts! Welcome back to another exciting coding session. Today’s problem is a treat—literally! We’re going to solve the “Design HashMap” or “LeetCode 706. ‘
- Initialization: We create a HashMap with 1,000 boxes (or arrays) to store our key-value pairs.
- Adding a Pair (Put): To add a key-value pair, we calculate the box (index) for the key and store it there. If the box is empty, we create a new shelf (linked list). If the key already exists on the shelf, we update the value. Otherwise, we place the pair on the shelf (linked list).
- Finding a Value (Get): To find a value, we calculate the box (index) for the key and look on the shelf (linked list). If the key is found, we return its corresponding value. If not, we return -1.
- Removing a Pair (Remove): Removing a pair is like taking a book off the shelf. We calculate the box (index), find the key on the shelf (linked list), and remove it.
Codes : Leet code 706.
C++:Leetcode 706.
#include <vector>
#include <list>
class MyHashMap {
private:
int size;
std::vector<std::list<std::pair<int, int>>> hash_map;
public:
MyHashMap() {
size = 1000;
hash_map.resize(size);
}
void put(int key, int value) {
int index = key % size;
for (auto& kv : hash_map[index]) {
if (kv.first == key) {
kv.second = value;
return;
}
}
hash_map[index].emplace_back(key, value);
}
int get(int key) {
int index = key % size;
for (const auto& kv : hash_map[index]) {
if (kv.first == key) {
return kv.second;
}
}
return -1;
}
void remove(int key) {
int index = key % size;
hash_map[index].remove_if([key](const std::pair<int, int>& kv) {
return kv.first == key;
});
}
};
Java: Leetcode 706.
import java.util.LinkedList;
class MyHashMap {
private int size;
private LinkedList<Pair>[] hash_map;
public MyHashMap() {
size = 1000;
hash_map = new LinkedList[size];
}
public void put(int key, int value) {
int index = key % size;
if (hash_map[index] == null) {
hash_map[index] = new LinkedList<>();
}
for (Pair pair : hash_map[index]) {
if (pair.key == key) {
pair.value = value;
return;
}
}
hash_map[index].add(new Pair(key, value));
}
public int get(int key) {
int index = key % size;
if (hash_map[index] == null) {
return -1;
}
for (Pair pair : hash_map[index]) {
if (pair.key == key) {
return pair.value;
}
}
return -1;
}
public void remove(int key) {
int index = key % size;
if (hash_map[index] == null) {
return;
}
hash_map[index].removeIf(pair -> pair.key == key);
}
private class Pair {
int key;
int value;
Pair(int key, int value) {
this.key = key;
this.value = value;
}
}
}
Python: Leet code 706.
class MyHashMap:
def __init__(self):
# Initialize an array with a predefined size (e.g., 1000)
self.size = 1000
self.hash_map = [None] * self.size
def put(self, key, value):
# Calculate the hash code for the key
index = key % self.size
# If the index is empty, create a new linked list
if self.hash_map[index] is None:
self.hash_map[index] = []
# Check if the key exists in the linked list
for i in range(len(self.hash_map[index])):
if self.hash_map[index][i][0] == key:
# Update the value if the key exists
self.hash_map[index][i] = (key, value)
return
# Append the (key, value) pair to the linked list
self.hash_map[index].append((key, value))
def get(self, key):
# Calculate the hash code for the key
index = key % self.size
# If the index is empty, return -1
if self.hash_map[index] is None:
return -1
# Search for the key in the linked list
for i in range(len(self.hash_map[index])):
if self.hash_map[index][i][0] == key:
return self.hash_map[index][i][1]
return -1 # Key not found
def remove(self, key):
# Calculate the hash code for the key
index = key % self.size
# If the index is empty, return
if self.hash_map[index] is None:
return
# Search for the key in the linked list and remove it
for i in range(len(self.hash_map[index])):
if self.hash_map[index][i][0] == key:
del self.hash_map[index][i]
return
Leetcode 1359 Count All Valid Pickup and Delivery Options (HARD)
JavaScript: Leet code 706.
class MyHashMap {
constructor() {
this.size = 1000;
this.hash_map = new Array(this.size);
}
put(key, value) {
const index = key % this.size;
if (!this.hash_map[index]) {
this.hash_map[index] = [];
}
for (const entry of this.hash_map[index]) {
if (entry[0] === key) {
entry[1] = value;
return;
}
}
this.hash_map[index].push([key, value]);
}
get(key) {
const index = key % this.size;
if (!this.hash_map[index]) {
return -1;
}
for (const entry of this.hash_map[index]) {
if (entry[0] === key) {
return entry[1];
}
}
return -1;
}
remove(key) {
const index = key % this.size;
if (!this.hash_map[index]) {
return;
}
this.hash_map[index] = this.hash_map[index].filter(entry => entry[0] !== key);
}
}
Resule Analysis
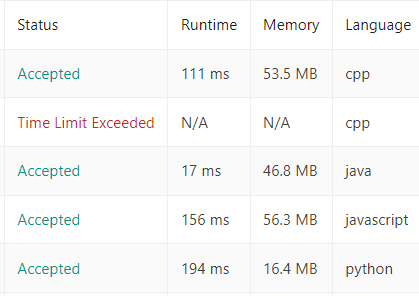