Leetcode 775. Find The Global and Local Inversions :Hey there, coding enthusiasts! Welcome back to another exciting coding session. Today’s problem is a treat—literally! We’re going to solve the “Global and Local Inversions ” problem.
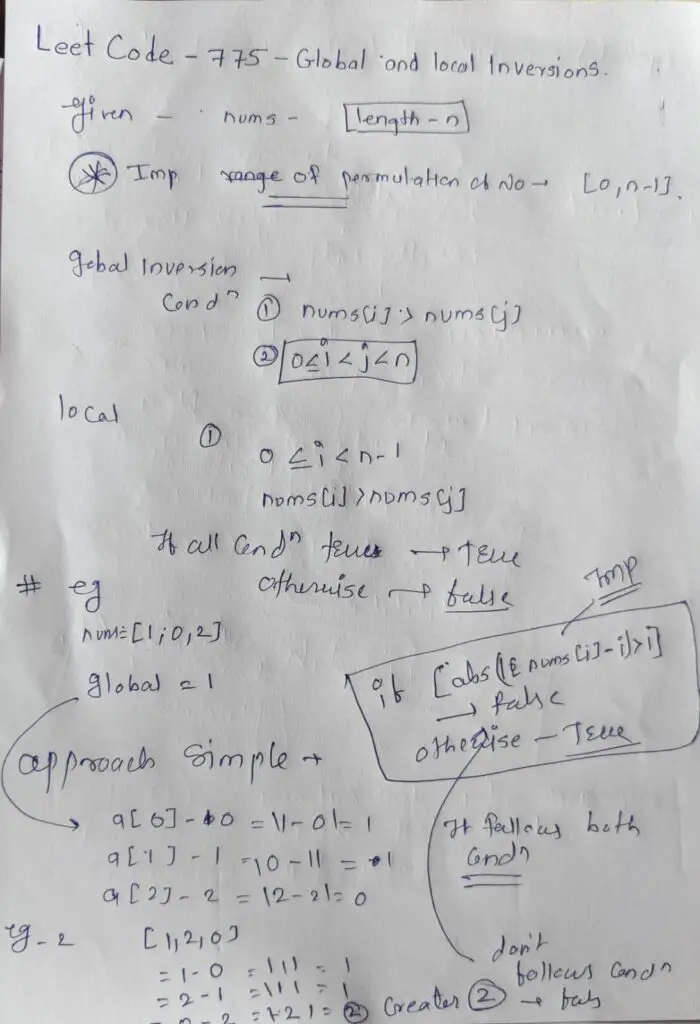
Leet code 775 Code Explanation: Global and Local Inversions
- 1: We’re given an array of numbers called
nums
. The goal is to determine if every “local inversion” is also a “global inversion.” - 2: A local inversion occurs when an element is larger than the next element.
- For example, in
[1, 3, 2]
, the pair3, 2
is a local inversion because3
is larger than2
.
- For example, in
- 3: A global inversion is when the number of local inversions equals the number of global inversions.
- For example, in
[1, 3, 2]
, there are 2 local inversions (3, 2
and1, 3
) and 2 global inversions (3, 2
and1, 3
).
- For example, in
- 4: The code iterates through each element in the
nums
array. - 5: For each element, it calculates the absolute difference between the element’s value and its index. If this difference is greater than 1, it means there’s a non-local inversion, so we return
false
. - 6: If we reach the end of the array without finding any non-local inversions, it means every local inversion is also a global inversion, and we return
true
.
Codes :
C++: Leetcode 775
#include <vector>
#include <cstdlib>
class Solution {
public:
bool isIdealPermutation(std::vector<int>& nums) {
for (int i = 0; i < nums.size(); ++i) {
if (std::abs(nums[i] - i) > 1) return false;
}
return true;
}
};
Leet Code : Validate Binary Search Tree Java | CPP | Python solution
Java:Leetcode 775
import java.util.*;
class Solution {
public boolean isIdealPermutation(int[] nums) {
for (int i = 0; i < nums.length; i++) {
if (Math.abs(nums[i] - i) > 1) return false;
}
return true;
}
}
Tackling Jump I , II , III , IV Game Problems on LeetCode | Cpp ,Java ,Python – Day 3
Python: Leetcode 775
class Solution:
def isIdealPermutation(self, nums: List[int]) -> bool:
for i in range(len(nums)):
if abs(nums[i] - i) > 1:
return False
return True
JavaScript: Leetcode 775
class Solution {
isIdealPermutation(nums) {
for (let i = 0; i < nums.length; i++) {
if (Math.abs(nums[i] - i) > 1) return false;
}
return true;
}
}
List of Some important Leet code Question :
- Leet Code 835. Image Overlap
- Leet Code 662. maximum width of binary tree
- Leet Code 287 Find the Duplicate Number
- Leetcode 135 Candy (Hard) Solution
- Leet Code 2612 Minimum Reverse Operations
- Leet code 206 Reverse Linked List
- Leet Code 420 Strong Password Checker
- Leetcode 1359 Count All Valid Pickup and Delivery
- Leet code 799. Champagne Tower
- Leetcode 775. Find The Global and Local Inversions